Learning Python programming for beginners can be an exciting journey, especially given its rising popularity and application in various fields. Python, known for its simplicity and readability, is an excellent starting point for aspiring programmers. In this guide, we’ll explore the essentials of Python programming, provide practical examples, and offer tips for mastering Python quickly and effectively.
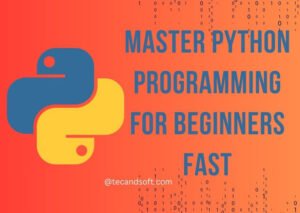
Introduction to Python Programming for Beginners
Python programming for beginners is designed to introduce new coders to the basics of Python, a versatile and powerful programming language. Python’s syntax is clear and easy to learn, making it an ideal first language. Its extensive libraries and community support further simplify the learning process.
Why Choose Python?
Python’s growing popularity is attributed to its versatility and user-friendly nature. It is used in web development, data analysis, artificial intelligence, scientific computing, and more. This broad applicability means that learning Python opens numerous career opportunities.
Setting Up Your Python Environment
To start with Python programming for beginners, setting up your environment is crucial. You can write Python code using various tools:
- Python Online Compiler: Ideal for quick coding and testing.
- PyCharm: A powerful Integrated Development Environment (IDE) for Python.
- W3Schools Python: Offers an interactive platform to practice coding.
Installing Python
Python is available for free on the official Python website. Download the latest version and follow the installation instructions for your operating system. Ensure that the PATH variable is set correctly to run Python from the command line.
Basic Concepts of Python Programming for Beginners
Understanding the fundamental concepts is key to mastering Python programming for beginners. Let’s explore some core elements:
Variables and Data Types
Python supports various data types, including integers, floats, strings, and booleans. Variables store data values, and their type is determined automatically based on the value assigned.
# Example of variable assignment name = “John”age = 25is_student = True
Control Structures
Control structures allow you to manage the flow of your program. Common structures include conditionals (if-else statements) and loops (for and while loops).
# Example of a conditional statementif age < 18: print(“Minor”)else: print(“Adult”) # Example of a for loopfor i in range(5): print(i)
Functions
Functions are reusable blocks of code that perform specific tasks. They help in organizing code and avoiding repetition.
# Example of a functiondef greet(name): return f”Hello, {name}!” print(greet(“Alice”))
Practical Python Programming Examples
To master Python programming for beginners, practice is essential. Let’s look at some practical examples:
Basic Calculator
A simple calculator that performs basic arithmetic operations.
def add(a, b): return a + b def subtract(a, b): return a – b def multiply(a, b): return a * b def divide(a, b): if b != 0: return a / b else: return “Error! Division by zero.” # User inputnum1 = float(input(“Enter first number: “))num2 = float(input(“Enter second number: “))operation = input(“Enter operation (+, -, *, /): “) if operation == ‘+’: print(add(num1, num2))elif operation == ‘-‘: print(subtract(num1, num2))elif operation == ‘*’: print(multiply(num1, num2))elif operation == ‘/’: print(divide(num1, num2))else: print(“Invalid operation”)
Fibonacci Sequence
Generating the Fibonacci sequence up to a specified number of terms.
def fibonacci(n): sequence = [0, 1] while len(sequence) < n: sequence.append(sequence[-1] + sequence[-2]) return sequence # User inputterms = int(input(“Enter number of terms: “))print(fibonacci(terms))
Advanced Topics in Python Programming for Beginners
Once you are comfortable with the basics, you can explore more advanced topics to enhance your Python programming skills further.
Object-Oriented Programming (OOP)
Python supports OOP, which helps in organizing code into classes and objects. This approach is beneficial for large projects.
# Example of a classclass Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): return f”{self.name} says woof!” # Creating an objectmy_dog = Dog(“Buddy”, 3)print(my_dog.bark())
Working with Libraries
Python’s vast array of libraries extends its functionality. Libraries such as NumPy for numerical computations, Pandas for data manipulation, and Matplotlib for data visualization are widely used.
# Example using NumPyimport numpy as np array = np.array([1, 2, 3, 4, 5])print(array * 2)
Tips for Learning Python Programming for Beginners
To master Python programming for beginners, consider the following tips:
Practice Regularly
Consistent practice is crucial. Work on small projects and gradually increase their complexity.
Join a Community
Engage with the Python community through forums, social media, or local meetups. This provides support and networking opportunities.
Use Online Resources
Utilize free online resources such as W3Schools Python tutorials and interactive coding platforms. These resources offer guided learning paths and hands-on practice.
Take a Python Course
Enrolling in a structured Python course can provide a comprehensive learning experience. Many online platforms offer free and paid courses tailored to different skill levels.
Experiment with Projects
Apply your knowledge by working on real-world projects. This not only reinforces your learning but also builds a portfolio that can impress potential employers.
Revolutionizing AI for Elderly Care Solutions
Python’s capabilities extend beyond traditional programming. One such example is revolutionizing AI for elderly care solutions. Python’s machine learning libraries, such as TensorFlow and PyTorch, enable the development of intelligent systems that can assist the elderly in daily tasks, monitor their health, and improve their quality of life.
Conclusion
Mastering Python programming for beginners is a rewarding endeavor that opens doors to various technological fields. By understanding the basics, practicing regularly, and exploring advanced topics, you can quickly become proficient in Python. Whether you are interested in web development, data science, or AI, Python provides the tools and community support to help you succeed.
#PythonProgrammingForBeginners #LearnPythonFree #PythonOnlineCompiler